There are a lot of excellent open source content management systems available today, WordPress being one of them, which is widely used. WordPress is a PHP based CMS so not a lot of cohesion is available with other technologies like Java, and is simply ignored as a viable content management option just for being non-Java.
In this post I will demonstrate how WordPress can be integrated with Spring Boot applications, all made possible with WordPress REST plugin and a Spring client. Both of these are open source and available for free. Below is a simple design diagram that explains how this integration works. This post assumes that the reader has prior knowledge of WordPress and Spring Boot.
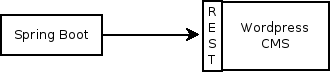
In the spring boot application add the following dependency in order to include the Spring WP-API client.
<dependency> <groupId>org.kamranzafar.spring.wpapi</groupId> <artifactId>spring-wpapi-client</artifactId> <version>0.1</version> </dependency>
Below is a sample configuration we need in the application.properties file, so that we can connect our Spring Boot application to our WordPress site.
wpapi.host=yoursite.com wpapi.port=443 # Optional, default is 80 wpapi.https=true # Optional, default is false
The Spring WP-API client needs the RestTemplate so lets create a bean in our Application and autowire the Spring client. We also need to add component scan annotation so that Spring finds and autowires all the required dependencies.
@SpringBootApplication @ComponentScan("org.kamranzafar.spring.wpapi") public class Application implements CommandLineRunner { public static void main(String args[]) { SpringApplication.run(Application.class); } @Autowired private WpApiClient wpApiClient; @Bean public RestTemplate restTemplate() { return new RestTemplate(); } }
Now our spring boot application is all setup to connect to out WordPress site in order to retrieve and create content. So lets try and lookup all the posts having the keyword “Spring”, below is how we can do this.
Map<String, String> params = new HashMap<>(); params.put("search", "Spring"); params.put("per_page", "2"); // results per page params.put("page", "1"); // current page // See WP-API Documentation more parameters // http://v2.wp-api.org/reference/ Post[] posts = wpApiClient.getPostService().getAll(params); for (Post p : posts) { log.info("Post: " + p.getId()); log.info("" + p.getContent()); }
The complete test application is available with the Spring WP-API client on Github.
This is just a small example of how we can integrate Spring Boot and WordPress. We can take this further by properly integrating WordPress in the Spring web applications platform, e.g. by enabling Single Sign On using the CAS plugin.
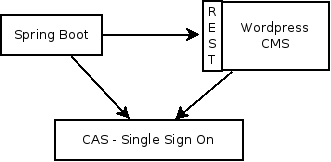
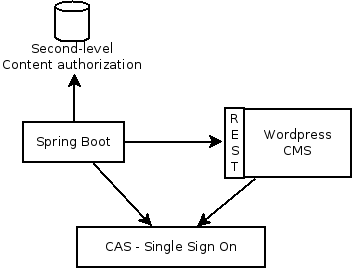
2 Comments
Hi Kamran,
Thank you for this article and sources’s project. Very helpull and usefull.
I’ve installed plugins in wordpress :
– WP REST API (to accept rest call)
– VA Simple Basic Auth
– WP OAuth Server
But, i still have 403 response (access denied)
What’s type of authentification do you use ?
How can i set it correctly in wordpress ? is it a problem of .htaccess ?
How can i log the requests in the project ?
Thank for your response.
[…] that uses WP REST API plugin to fetch content from WordPress. You might find it useful. Here is blog post on how to integrate Spring Boot and […]